How to Do Integration Testing With React
•10 min read
- Languages, frameworks, tools, and trends
- Skills, interviews, and jobs
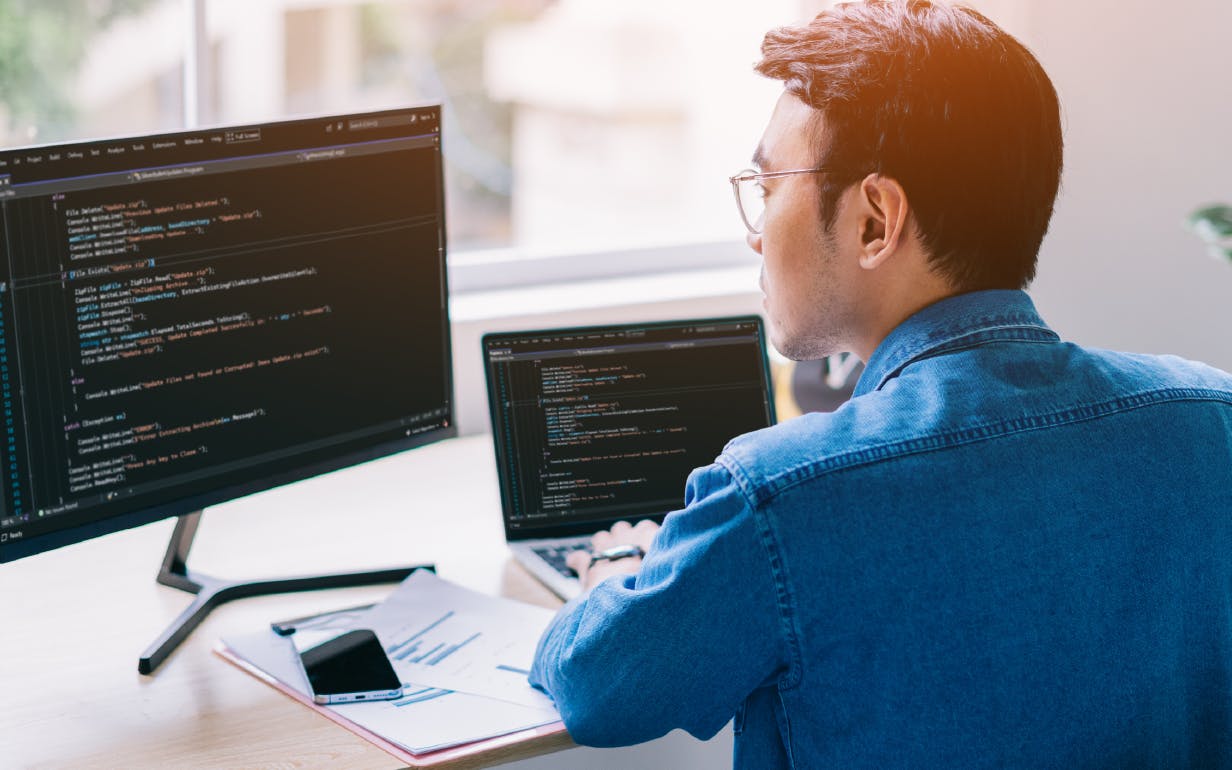
Software development is incomplete without integration testing. It is particularly important if you work with many third-party apps or APIs in your React application. You need to ensure that everything works well together and nothing breaks when you upgrade to the newest version.
Setting up the right tests for React components can be a pain. This could result in false positives and negatives if it's not done correctly. Running integration tests for your React application can be tricky as well.
Here you will get the exact steps on how to set up the project, write tests, and run them through the process of development.
Let's start by defining what is meant by an integration test and see how it is different from unit testing and functional testing.
What is integration testing?
A second level of a software testing process, right after the unit testing, is the Integration testing. In this type of testing, different parts or components of the software are tested in conjunction. Integration testing is focused on catching flaws in interactions between integrated units or components.
The testing combines modules that are tested as part of unit testing. Different coders or programmers work on different software modules that make up the whole software. Integration testing checks whether all the modules are properly communicating with each other.
Integration testing is a type of software testing that verifies how individual software modules or groups of modules interact with each other. These tests ensure that the overall system works correctly in terms of interactions between software components. As soon as all the components or modules are independent of each other, we need to check the flow of data between them. And this test is conducted using integration testing.
For interactive websites, like those built with React, integration tests are a natural fit. This category test validates how a user interacts with your application without the adding overhead of an end-to-end test.
Three types of tests that are commonly get conducted:
- Unit testing verifies a single piece of code in isolation at a time. However, this does not cover the big picture.
- Testing from end-to-end (E2E), on the other hand, uses automation tools - such as Selenium or Cypress - to play like a user on your website: filling in forms, clicking buttons, etc. While they generally take longer to write and run, the user experience gets closely matched.
- An integration test is somewhere between these two. It verifies how your application works together across multiple units but is smaller and lighter than E2E tests. With Jest, for example, integration testing is made easier with several built-in tools, namely jsDOM for emulating browser APIs with minimal overhead and mocking tools for simulating external APIs.
Benefits of integration testing with React
As discussed earlier, the three most common types of tests are unit, integration, and end-to-end testing.
Among them, there are plenty of different ways to do integration tests. For example, you can test how your components work together, and you can mock certain things (such as HTTP requests), so they're not too time-consuming to write. Plus, they give a lot of confidence in your work. By starting to test your components this way, you will not need as many unit tests at the end of the day.
This is the reason you should spend your time writing integration tests. However, you can also write unit tests and end-to-end tests that are also valuable.
Integrating tests provide you with an ideal balance between confidence and effort, so it's wise to spend most of the time writing them.
Testing React apps - common approaches
The four primary integration testing methods involve big-bang testing, top-down testing, bottom-up testing, and sandwich/hybrid testing. Each method has its advantages and disadvantages and we will discuss them all in detail.
Big-bang testing
As part of the big-bang approach, all modules must be integrated together and tested as a single unit. The Big Bang Integration testing practice involves connecting all units at the same time and producing a complete system in the end.
The problem with this kind of testing strategy is that it is difficult to isolate any identified errors since interfaces between individual segments are not thoroughly tested.
Advantages
- Due to its suitability, small systems can be easily tested.
- By identifying errors in such systems, you can save time and speed up the application deployments.
Disadvantages
- It can be difficult to find the source of defects as different modules are integrated as one.
- When a large system has numerous units, big-bang testing takes a lot of time to generate output.
- Integration testing is a long process. And even the developers take longer to fix errors since all modules must be available before the testing procedure begins.
Top-down testing
Tests are performed incrementally from the topmost to the lowest level modules using the top-down approach. Testing for each component is done one by one, then the components are integrated to see how it functions as a whole.
- The process of identifying defects and isolating the causes is much easier.
- A test will find critical design flaws more easily if it checks important units first.
- Early prototypes can be created.
- The result of examining the lower-level modules can result in inadequate or incomplete testing.
- The testing process becomes complicated when there are too many testing stubs.
Bottom-up testing
The opposite of top-down integration testing is bottom-up integration testing (also called bottom-to-top testing). This method tests the lower-level modules first, and then gradually moves to the higher-level modules. It is suitable for testing all units when they are all available.
- Identifying and localizing faults are easier.
- Since no modules are waiting for testers to test, troubleshooting takes lesser time.
- Testing all modules can take a long time, which can lead to delay in the release of final product.
- Since testers only test critical modules in the last stages, some defects may be missed and developers may not have time to fix them.
- A software system with multiple low-level units can make testing more challenging.
- Creating an early prototype is not possible with this method.
Sandwich/hybrid testing
Testing integration refers to the process of combining and testing separate modules of software into one entity. Testing integration is divided into two phases: interface and communication. integration testing is done both from top to bottom and from bottom to top approach.
Hybrid integration testing, also known as sandwich testing, combines both bottom-up testing and top-down testing methods.
Sandwich testing provides testers with both of these advantages, making the process more precise and efficient. A combination of stubs and drivers is used.
Advantage
- The most appropriate method for testing large programs, such as operating systems and other long-term programs.
- The process is complex and expensive.
- For this approach, a higher degree of precision and skill is required.
How should Integration testing be conducted?
Integration testing consists of the following steps:
- Developing a test plan.
- Designing test scenarios, test cases, and test scripts.
- Running tests after integration of units or modules.
- Reporting errors and fixing them.
- Retesting functionalities after bug fixes.
- Repetition of this process until all issues are resolved.
Introduction to Jest and React testing library
What is Jest?
Designed for JavaScript and TypeScript, Jest is a testing framework for JavaScript code. The framework integrates well with React.
It ensures the correctness of any JavaScript codebase by means of a testing framework. With it, you can write tests with a familiar, easy-to-use API that provides results quickly. With this well-documented, easy-to-configure framework, you can customize it to meet your requirements.
With its simplified design and powerful API for isolated tests, comparisons, mocking, test coverage, and much more, it is a framework that has been designed keeping simplicity in mind.
What is React testing library
Testing React components are made easier with React Testing Library, a JavaScript testing tool. It assures that the user interface behaves correctly by simulating user interactions on isolated components.
The React Testing Library was created by Kent C. Dodds and is extremely lightweight. This component provides light utility functions on top of react-dom and react-dom/test-utils, replacing the Enzyme component.
As a DOM testing library, React Testing Library tests DOM elements instead of rendered React components, which means it makes use of real-world DOM elements as opposed to React components.
You can easily get started with this as it promotes good testing practices, and it is (relatively) easy to use.
Getting started with the sample application
For creating the react app, you will have to have node.js installed on your machine because we will be using npm (node package manager).
Following this step, you can create a directory containing an src folder using the following command in the terminal. All the files associated with the UI of the application will be collected in this command.
We will begin by installing the required libraries and setting up the project. A React application can be easily set up and run with the command.
create-react-app.
It is recommended that you run the commands and write the code yourself while following the tutorial.
First, create a React app:
npx create-react-app tutorial
Now, install React Testing Library:
npm install --save-dev @testing-library/react
Finally, install additional libraries:
npm install axios
Type the following command in order to begin using the application. Your web browser will open up the react application. And with that your react application is created!
npm start
You can see the results in the browser by adding the following elements to the app.js file to fully understand the testing process.
In the app.js file, insert the following code for a small change to the existing React app, "This is My First React Application".
import React from 'react';
import './App.css';
function App() {
return (
<h1> This is My First React Application </h1>
);
}
export default App;
Output
Writing Integration Tests
import { render, screen } from '@testing-library/react';
import App from './App';
import '@testing-library/jest-dom'
describe('Checks the title component', () => {
it('checks the value of the Title component', () => {
const { getByText } = render(<App />);
const titleValue = getByText('This is My First React Application')
expect(titleValue).toBeInTheDocument('This is My First React Application')
})
})
Output
As you can see in the above image, the test suite has passed the test.
Jest and the React testing library manage component testing and unit testing respectively, but they cannot handle end-to-end testing, that is where the application must be tested to see how the components behave together.
It can be a daunting task to try to understand more advanced applications with multiple shortcomings in the way. For end-to-end testing of React-based applications, there are lots of tools available in the market that are quite helpful. Additionally, automated testing can also render better and faster results.
So, we've reached the end of our tutorial about integration testing with React. You can test everything now and you know how to do it in a way that fits into the React testing ecosystem.
Integration testing is the process in which we can test the integration between components. We can also do regression testing to check whether the changes are breaking anything or not.
It allows the developer to verify the full stack of an application. Apart from that everything works independently, integration tests are designed to examine the interactions between various parts of your development ecosystem.
Integration testing is a great way to check whether different components of your app work together as intended. In order to write integration tests, you can also use any framework. Most frameworks support testing React apps with visual representation.
FAQs
1. Is Jest good for integration testing?
Jest is an open-source test framework by Facebook that gets used for integration testing. Tests can be performed using a command-line tool similar to those used in Jasmine and Mocha. Not only UI testing, but also you can test the whole tech stack with the help of this framework.
It requires zero configuration to create mock functions. In addition to that, it provides a great feature called "snapshot testing." That helps the tester to check component rendering results.
2. Which tool allows you to test React apps?
For testing a React app, there are plenty of open-source tools available in the market. However, every available tool is different from other tools. Most developers choose Jest, Enzyme, or other tools for React component testing.
You can use these tools for unit testing and integration testing. Following is the list of some popular tools that most developers along with testers prefer to use for testing purposes.
- Jest
- Mocha
- Jasmine
- Enzyme
- Chai
- CypressIO
3. Which is better, Jest or Mocha?
Both Mocha and Jest are the best choice to perform all kinds of testing on React apps. Jest prefers a simple and straightforward approach for testing, while Mocha provides a few extra out-of-the-box features.
If you prefer easy testing without learning new syntax, Jest is the better choice for you. But if you want advanced features and powerful tools, you can try Mocha.
4. What is the difference between Jest and React testing library?
Jest is a test runner, so the tester prefers Jest to find tests, run the tests, and derive the test result whether it passed or failed. While React Testing Library prefers providing a virtual DOM for react component testing.
React testing allows to integrate with any other testing library, while Jest is a special tool and preferred by many developers
5. Can we use Jest without Enzymes?
Both Jest and Enzyme preferred to test the React application. Jest is flexible so you can use it with any other testing framework, but Enzyme only supports react.
You can use Jest without Enzyme, and testing can be done perfectly. But using Enzyme with Jest will add extra features to it. You can run Enzyme without Jest, but for that to work you have to use another test runner with it. In other words, Enzyme needs a test runner to perform testing