Python Multiprocessing vs Multithreading
•7 min read
- Software comparisons
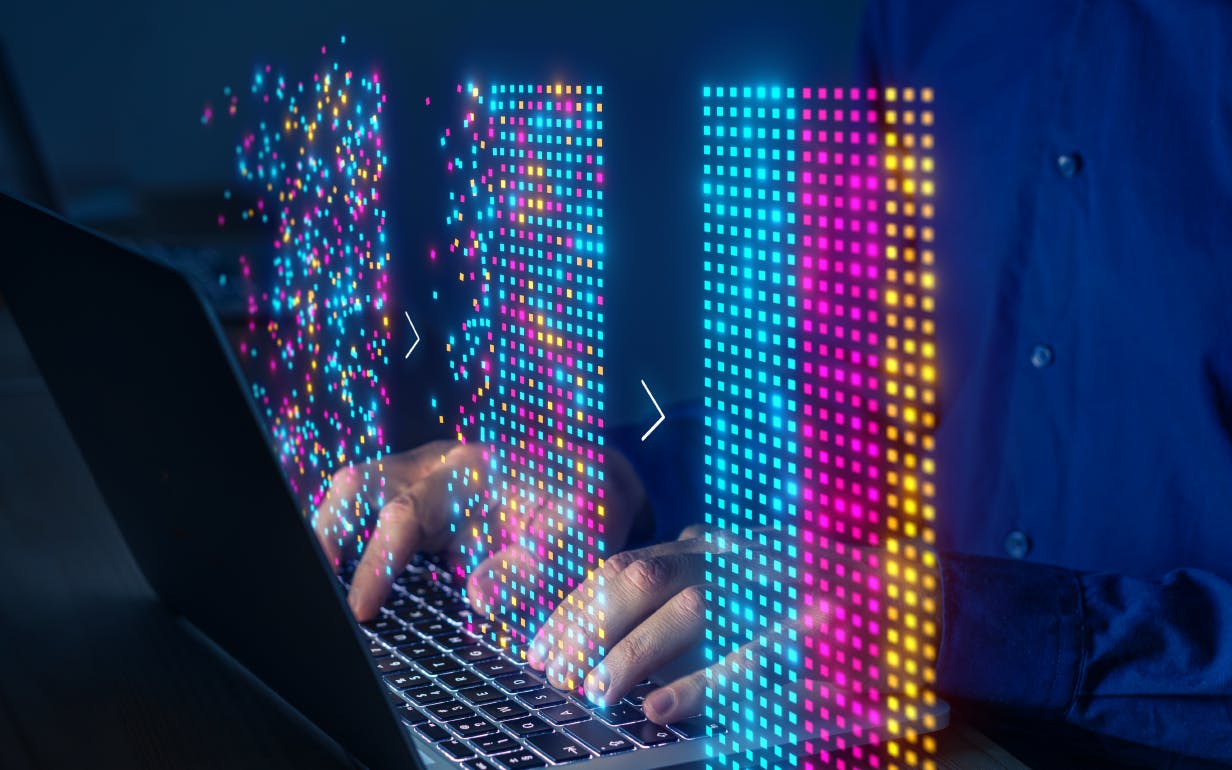
Humans are not built to efficiently multitask, and this is where machines come into the picture. In this article, we will discuss the tips and tricks that reduce time and increase performance in machines. We will walk you through Python multiprocessing vs multithreading, and how and where to implement these approaches.
What is a thread?
A thread is an independent flow of execution. It is synonymous with lightweight processes. It can be seen as an instance of an individual process.
In simpler terms, a thread is a sequence of instructions that a machine performs. It can also be preempted and temporarily interrupted by the processor depending on the scenario.
In general processes, a thread can be interrupted by the computer according to the situation. But in the case of Python 3, the threads appear to be executing simultaneously.
In software development, a thread simply means a task that often paves the way for Python developers to streamline the concurrency of the programs. This leads to a phenomenon known as multithreading.
What is multithreading in Python?
Multithreading is a task or an operation that can execute multiple threads at the same time.
To better understand the concept of multithreading in Python, we can use the following modules Python offers:
- Thread module: A thread module is an entirely separate execution flow. It streamlines multiple executions taking place at once.
- Threading module: The threading module offers an intuitive API for generating multiple threads that can be used to perform multithreading in Python.
Multithreading is a widely popular technique that streamlines multiple processes in quick succession at the same time. It facilitates the sharing of resources and data spaces of multiple threads with the main thread. This enables easy and efficient communication between different threads.
The image below explains multithreading in Python.
Python is a linear language. However, the threading module is useful when you need more processing power. Note that while multithreading in Python is the perfect choice for I/O operations and tasks, it cannot be used for web scraping processes as the processor waits for data and sits idle.
Multithreading is a game-changing technique as many scripts that are related to the I/O operations spend the majority of their time waiting for the data from a remote source. Because the downloads might not be linked, the processor can download from different data sources in parallel and combine the result at the end.
However, there is little benefit to using the threading module. The multithreading technique breaks down the processes into smaller fragments that run independently. The more tasks a single processor has, the more it becomes difficult for the processor to keep track of them.
Multithreading is included in the standard library.
You can use the target as the object that can be called. Apart from that, you can have ‘args’ to pass parameters to the function.
Let’s understand the concept better with an example. In the code below, we will learn how to perform mathematical calculations.
Code fragment:
Output:
In the above example, we have seen how to perform simple operations like finding out a square or cube of a number.
Locking variables
You will want threads to be able to modify the variables that are common between threads. To do this, you will need to use a lock that locks the variable it wants to modify. When another function wants to use a variable, it waits until that variable gets unlocked.
Let’s take an example to better understand this concept.
Let’s take two functions that iterate a variable by 1. The lock allows the developer to ensure that one function can perform the following operations.
- The function can access the variable.
- The function can write back to the variable before another function can access it.
- The function can perform calculations.
You may face issues with the text getting jumbled up and this can cause data corruption when incorporating multithreading. Thus, it is advisable to use lock to ensure that only one thread can be printed at a time.
Here’s an example to better understand the concept of lock. We have taken 5 workers who will complete 10 jobs.
Code:
Why is multithreading not always an option?
Multithreading streamlines different tasks, but the technique also comes with a few disadvantages. Here is why it is not always an option.
- There are a few overheads associated with managing multiple threads and you would not use multithreading for basic tasks like in the example above.
- While multithreading simplifies tasks, it can make debugging more difficult and increase the complexity of the program.
This brings us to the question: Does Python multithreading have a better alternative?
What is Python multiprocessing and how is it different to Python multithreading?
Multiprocessing is the ability of a processor to execute several unrelated processes simultaneously. These processes are independent and do not share any resources. Multiprocessing fragments multiple processes into routines that run independently. This ensures that every processor gets its own core for smooth execution.
Python programs are unable to max out your system’s specifications because of the global interpreter lock (GIL) without implementing the Python multiprocessing technique. The GIL is necessary as Python is not thread-safe.
Multiprocessing in Python is an effective mechanism for memory management. It enables you to create programs that bypass the GIL and make optimum use of your CPU core. Although the process is different from the threading library, the syntax is quite similar. The Python multiprocessing library gives each process its own Python interpreter and GILs.
Multiprocessing eliminates the issues associated with threading such as deadlocks and data corruption. Apart from that, the processes cannot modify the same memory as they do not share them.
Getting started with Python multiprocessing
Here’s an example to better understand Python multiprocessing.
If the database you use is a shared one, you might want to ensure that you wait for the relevant processes to finish before starting with the new ones.
Just like we saw in Python multithreading, you can pass arguments to your program using ‘args’.
Python multiprocessing vs multithreading
If your program is IO-bound, both multithreading and multiprocessing in Python will work smoothly. However, If the code is CPU-bound and your machine has multiple cores, multiprocessing would be a better choice.
Here is a detailed comparison between Python multithreading and multiprocessing.
When to use multithreading in Python?
Multithreading would be the best choice if you want to fragment your tasks and operations into multiple sub-tasks and then execute them simultaneously. With proper multithreading in place, you can improve these important aspects:
- Time consumption
- Speed
- Rendering
- Performance
Advantages of Python multithreading
Multithreading in Python offers many advantages that make it a good choice and a widely popular approach. Here are the two main advantages:
- Multithreading in Python streamlines the efficient utilization of resources as the threads share the same memory and data space.
- It also allows the concurrent appearance of multiple tasks and reduces the response time. This improves the performance.
Does Python support multithreading?
In the case of parallel computing, Python does not support multithreading. For tasks that require parallel computation, you should consider multiprocessing.
Any task or program that uses a pure Python code and tries to get a speed boost from parallel execution will not see any increase in speed as the threaded Python code is locked to one thread that executes at a time. However, in the case of NumPy or PIL operations, any C code can run in parallel with one active Python thread.
Python multithreading works great for creating a responsive graphic user interface and for handling short web requests where one thread handles the GUI actions and the other processes the files one at a time.
Now that you’ve learned the workings of Python multiprocessing and multithreading as well as how they stack up against each other, you can write code efficiently and implement the two techniques in different situations.
FAQs
Why is multithreading not possible in Python?
Python does not support multithreading as the CPython interpreter does not support multi-core execution through multithreading. It will not allow you to use the extra CPU cores.
How do I speed up my Python code?
Most Python functions are written in C, which is why they are faster than a pure Python code. You can incorporate summing of numbers and loop through each as you go. Here are a few ways to speed up your Python code:
- Use the While Loop
- Use a tuple
- Avoid global variables
- Use generators to save time
- Use 1 for infinity loops to speed up the runtime
- Use NumPy, SciPy, and Pandas for large datasets