How to Use Python Language for Server Side Language
•6 min read
- Languages, frameworks, tools, and trends
- Skills, interviews, and jobs
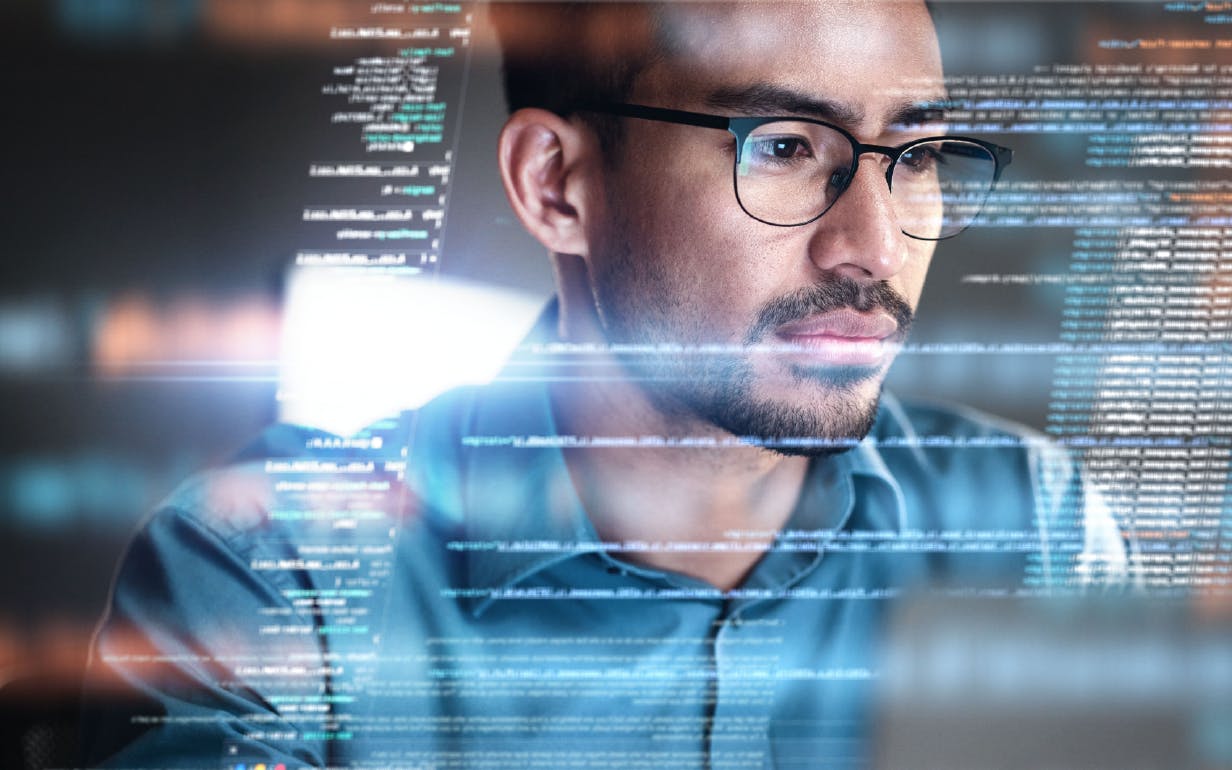
Python is one of the best programming languages to learn with applications in back-end web development, machine learning and statistics, scientific modeling, system operations, and several enterprise-specific software. Due to its English-like syntax, dynamic typing, and many libraries, it’s generally considered one of the more approachable programming languages.
This article will explore Python for server-side programming.
First, a quick look at what a server is.
Image source: sites.nd.edu
The server is a client-server model in the infrastructure of the internet. Whenever a client browser visits a web page, it generates an HTTP request to the server holding the files required to manage a website. The server attends to the client’s request and processes it, responding with the necessary information to present the web page.
Server-side programming is classified into static and dynamic web servers. The former returns files as they are while the latter introduces databases and application servers.
Note: ‘File not found’ or 404, a ‘famous’ server error code that sometimes occurs when browsing the internet, is due to the server being unable to access some files.
Starting a web server in Python
Launching a Python web server takes only a few minutes as it’s quick and straightforward. Just one line of code gets the simplest local server running on a system. Through local testing, the system becomes the server to the client, which is the browser. The files are stored locally on the system.
To create a web server, you will need a Python HTTP server module that can be used as a static file server. For a dynamic web server, you will need a Python framework like Django or Flask.
The code:
python -m http.server
Type the code above into your command prompt or terminal, depending on your system. The ‘server started’ message will appear. And there you have it - your first Python webserver.
This is admittedly a simple code that does little more than open up a web server on your system’s default port of 8000. The port can be changed by specifying the port number at the end of the line.
python -m http.server 8080
Python code:
Image source: by hacker.io
Dynamic web servers
Dynamic server-side websites deliver customized information in response to HTTP requests. They fulfill many operations such as retrieving data from a database, showing it on a page, validating user-entered data, saving it in a database, etc. The hugely popular Django is a completely featured dynamic server-side web framework written in Python.
Consider the number of products available in online retail stores and the number of posts written on social media. Displaying all of these using different static pages would be inefficient. So, instead of showing static templates (built using HTML, CSS, and JavaScript), it’s better to dynamically update the data displayed inside those templates when needed, such as when you want to view a different product in a retail store.
A brief overview of Django
Django is a high-level Python server-side web framework that allows the fast development of maintainable and protected websites. The open-source and free framework eliminates much of the hassle of web development. It also has a documented active community that provides many options at no cost.
Features of Django
- Secure: Django provides a framework that’s engineered to ‘do the right things’ to automatically protect websites. For example, it provides a secure way to manage user accounts and passwords.
Django can inspect if an entered password is correct by running it through a cryptographic hash function and resembling the output to the stored hash value. Due to this ‘one-way’ method, even if a stored hash value is compromised, it’s hard for an attacker to work out the original password. As it is, Django enables protection against many vulnerabilities by default, including SQL injection, cross-site scripting, cross-site request forgery.
- Versatile and complete: Django obeys the ‘batteries included’ philosophy by providing nearly everything that developers need to do things out-of-the-box. It helps create websites that include content management design systems, wikis to special networks, and news sites. It can also work in any client-side framework and delivers content in almost any format, such as HTML, JSON, XML, etc.
- Scalable: Django utilizes a component-based ‘shared-nothing’ architecture, which means that each part of the architecture is independent of the others. This allows it to be replaced or changed as required. The clear separation enables it to be scaled for increased traffic by adding hardware at any level, be it caching servers, database servers, or application servers.
- Maintainable and portable: Django code is written in such a way that it is reusable and maintainable. It follows the Don't Repeat Yourself (DRY) principle. There is no unnecessary duplication, which reduces the amount of code. It promotes grouping of related functionality into reusable ‘applications’, and at a lower level, groups related code into modules along with the line of the model-view-controller (MVC) pattern.
As Django is written in Python and runs on many platforms, you are not tied to any particular server platform. You can run applications on many flavors of Linux, Windows, and Mac OS X. What’s more, it’s well-supported by many web hosting providers that often provide specific infrastructure and documentation for hosting Django sites.
Django workflow
Traditional data-driven websites observe the following workflow:
- Web applications wait for the HTTP request from the web browser.
- Once the request receives the application, they work out what is needed based on the URL and possibly information in POST data or GET data. Then, depending on the requirements, they read or write data from a database or other tasks that need to satisfy the request.
- The applications return a response to the web browser in the form of HTML templates.
Common terminology
- URL: A mapper that maps the HTTP request from the client-side and redirects to the appropriate view based on the request URL. The mapper matches the particular patterns of strings and digits present in the URL.
- View: Acts as a request handler function that receives HTTP requests and returns HTTP responses.
- Models: Python objects that define the structure of an application's data and provide mechanisms to manage (add, modify, delete) and query records in the database.
- Template: A text file defining the structure or layout of a file (such as an HTML page), with placeholders used to represent actual content. A view can dynamically create an HTML page using an HTML template, populating it with data from a model.
Python code to implement workflow of Django
Sending the request (urls.py)
Typically, the urls.py file is stored in the URL mapper.
url_patterns = [
path("admit url"),
path('turing/<int:id>/', views.turing_detail, name='turing_detail'),
path('catalogs/', include('catalogs.urls')),
re_path(r'^([0-9]+)/$', views.best),
]
url_patterns is a mapper that defines a list of mappings between the specific URL_pattern and the corresponding view function. If the URL matches a certain pattern, the associated view function will be called and pass the request.
Handling the request (views.py)
Views are the heart of the web application, receiving HTTP requests from web clients and returning HTTP responses.
from django.http import HttpResponse
def index_1(request):
# Get an HttpRequest - the request parameter
# perform operations using information from the request.
# Return HttpResponse
return HttpResponse(“Hello, welcome to turing.com”')
Defining data models (models.py)
The data model helps Django web applications handle and query data through Python objects. Models give the structure of stored data, which includes field types, maximum size, default values, selection list options, text for documentation, etc.
from django.db import models
class Team(models.Model):
team_name = models.CharField(max_length=40)
TEAM_LEVELS = (
('U09', 'Under 09s'),
('U10', 'Under 10s'),
('U11', 'Under 11s'),
... #list other team levels
)
team_level = models.CharField(max_length=3, choices=TEAM_LEVELS, default="U11")
Rendering data (HTML templates)
Template systems authorize you to determine the structure of an output document by using placeholders for data that will be filled in when a page is generated. Templates are usually used to create HTML but can also create other types of documents.
##filename: best/templates/best/index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Home page</title>
</head>
<body>
{% if youngest_teams %}
<ul>
{% for team in youngest_teams %}
<li>{{ team.team_name }}</li>
{% endfor %}
</ul>
{% else %}
<p>No teams are available.</p>
{% endif %}
</body>
</html>
Many large-scale websites use server-side programming to display data dynamically whenever required. The biggest benefit of server-side code is that it permits you to tailor website content for various users. It has complete access to the server operating system, and can be written in different programming languages like Python, PHP, Ruby, C#, and JavaScript (NodeJS).