Chat Application via Python: A Complete Guidebook
•5 min read
- Languages, frameworks, tools, and trends
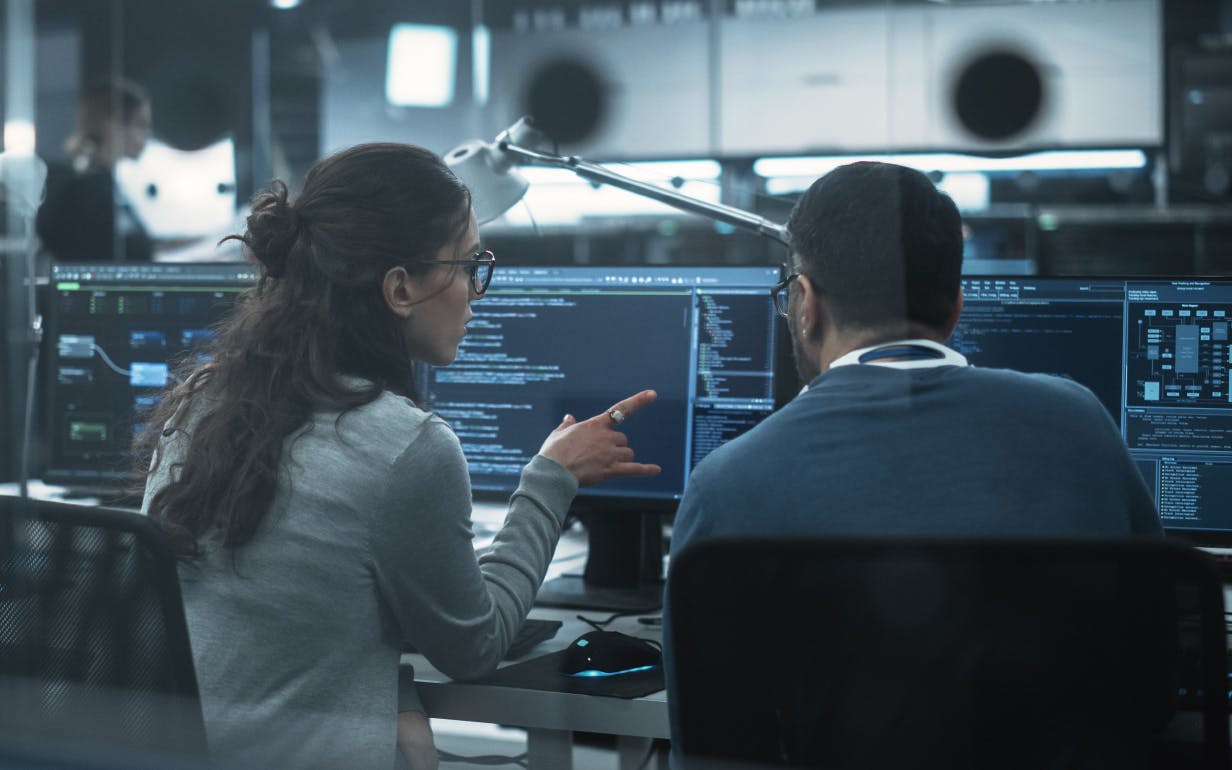
A chatbot is a computer program that is designed to simulate a human conversation. Chatbot, a natural language processing (NLP) tool, is typically used by Python developers in online customer service to help users with simple tasks such as setting up an account, making a purchase, finding a product, enquiring about returns and refunds, etc. In 2019, chatbots were able to handle nearly 69% of chats from start to finish - a huge jump from the year 2017 when they could process just 20% of requests.
There are many ways to create a chat application in Python. One is to use the built-in module called threading, which allows you to build a chatbox by creating a new thread for each user. Another way is to use the "tkinter" module, which is a GUI toolkit that allows you to make a chatbox by creating a new window for each user.
Types of chatbots
Chatbots can be categorized into two primary types:
- Rule-based chatbots
- Self-learning chatbots, under which there are retrieval-based chatbots and generative chatbots.
Rule-based chatbots
A rule-based chatbot is one that relies on a set of rules or a decision tree to determine how to respond to a user's input. The chatbot will go through the rules one by one until it finds a rule that applies to the user's input. It will then respond according to the rule.
Rule-based chatbots are often employed in customer service applications where they can be used to automate simple tasks such as providing information about a product or answering common customer service questions. They can also be used in games to provide hints or walkthroughs.
Self-learning chatbots
A self-learning chatbot uses artificial intelligence (AI) to learn from past conversations and improve its future responses. It does not require extensive programming and can be trained using a small amount of data.
Self-learning chatbots are an important tool for businesses as they can provide a more personalized experience for customers and help improve customer satisfaction.
- Retrieval-based chatbots: Retrieval-based chatbots are designed to retrieve predefined responses to user queries. They are not designed to generate new responses. The bot is trained using a collection of predefined responses to user queries. When a user asks a question, the bot searches its collection of predefined responses for the one that best matches the user’s question.
- Generative chatbots: Generative chatbots are designed to generate new responses to user queries and not to retrieve predefined responses. The bot is trained using a collection of predefined responses to user queries. When a user asks a question, the bot generates a new response by combining the predefined responses in its collection.
Steps to create a chatbot using Python
1. Setting up application dependencies
The process of building a chatbot in Python begins with the installation of the ChatterBot library in the system. For best results, make use of the latest Python virtual environment.
Use the following command in the Python terminal to load the Python virtual environment.
pip install chatterbot
pip install chatterbot_corpus
You can also upgrade the command with:
pip install --upgrade chatterbot_corpus
pip install --upgrade chatterbot
2. Importing classes
The next step is to import the classes into your system. There are two classes that are required, ChatBot and ListTrainer from the ChatterBot library.
Use the following command to import the classes:
from chatterbot import ChatBot
from chatterbot.trainers import ListTrainer
3. Creating and operating the chatbot
Now that you have imported the relevant classes, it’s time to create an instance of the chatbot, which is an instance of the class "ChatBot". Once you create a new ChatterBot instance, you need to train the bot to make it more efficient. The training will aim to supply the right information to the bot so that it will be able to return appropriate responses to users.
Use the following command to train the chatbot:
my_bot = ChatBot(name='PyBot', read_only=True,
logic_adapters=
['chatterbot.logic.MathematicalEvaluation',
'chatterbot.logic.BestMatch'])
Here, the value that passes in the function corresponds to the parameter name, which is the name of the Python chatbot. If you want to disable the bot’s learning ability after the training, add the "read_only=True" command in the function. The command "logic_adapters" means the list of adapters involved to train the chatbot.
The objective of the "chatterbot.logic.MathematicalEvaluation" command helps the bot to solve math problems. The "chatterbot.logic.BestMatch" command enables the bot to evaluate the best match from the list of available responses.
Note that you need to supply a list of responses to the bot. You can also do it by specifying the lists of strings that can be utilized for training the Python chatbot, and choosing the best match for each argument.
Here’s one such example of responses you can use to train the chatbot in Python:
You can also develop and train the chatbot using an instance called "ListTrainer" and assign it a list of similar strings.
The chatbot has now been enabled for communication.
4. Communicating with the chatbox
To interact with the chatbot, use the .get_response() function. It will appear as follows during the communication:
>>> print(my_bot.get_response("hi"))
how do you do?
print(my_bot.get_response("i feel awesome today))
excellent, glad to hear that.
>>> print(my_bot.get_response(what's your name?"))
i'm pybot. ask me a math question, please.
>>> print(my_bot.get_response(show me the Pythagorean theorem"))
a squared plus b squared equals c squared.
>>> print(my_bot.get_response("do you know the law of cosines?"))
c^2 = a^2 + b^2 - 2ab cos(gamma)
Keep in mind that the chatbot will not be able to understand all the questions and will not be capable of answering each one. Since its knowledge and training input is limited, you will need to hone it by feeding more training data.
5. Training the chatbot with corpus of data
The last process of building a chatbot in Python involves training it further. To do this, you can leverage existing corpus of data.
An example of training your Python chatbot is offered by the bot itself:
from chatterbot.trainers import ChatterBotCorpusTrainer
corpus_trainer=ChatterBotCorpusTrainer(my_bot)
corpus_trainer.train('chatterbot.corpus.english')
The best part about ChatterBot is that it provides such functionality in many different languages. You can also select a subset of a corpus in whichever language you prefer.
There is more than one way to make a chatbot in Python. For instance, you can use libraries like spaCy, DeepPavlov, or NLTK that allow for more advanced and easy-to understand functionalities. SpaCy is an open source library that offers features like tokenization, POS, SBD, similarity, text classification, and rule-based matching. NLTK is an open source tool with lexical databases like WordNet for easier interfacing. DeepPavlov, meanwhile, is another open source library built on TensorFlow and Keras.