How to Write Clean Codes by Using Pipe Operations in Python?
•6 min read
- Languages, frameworks, tools, and trends
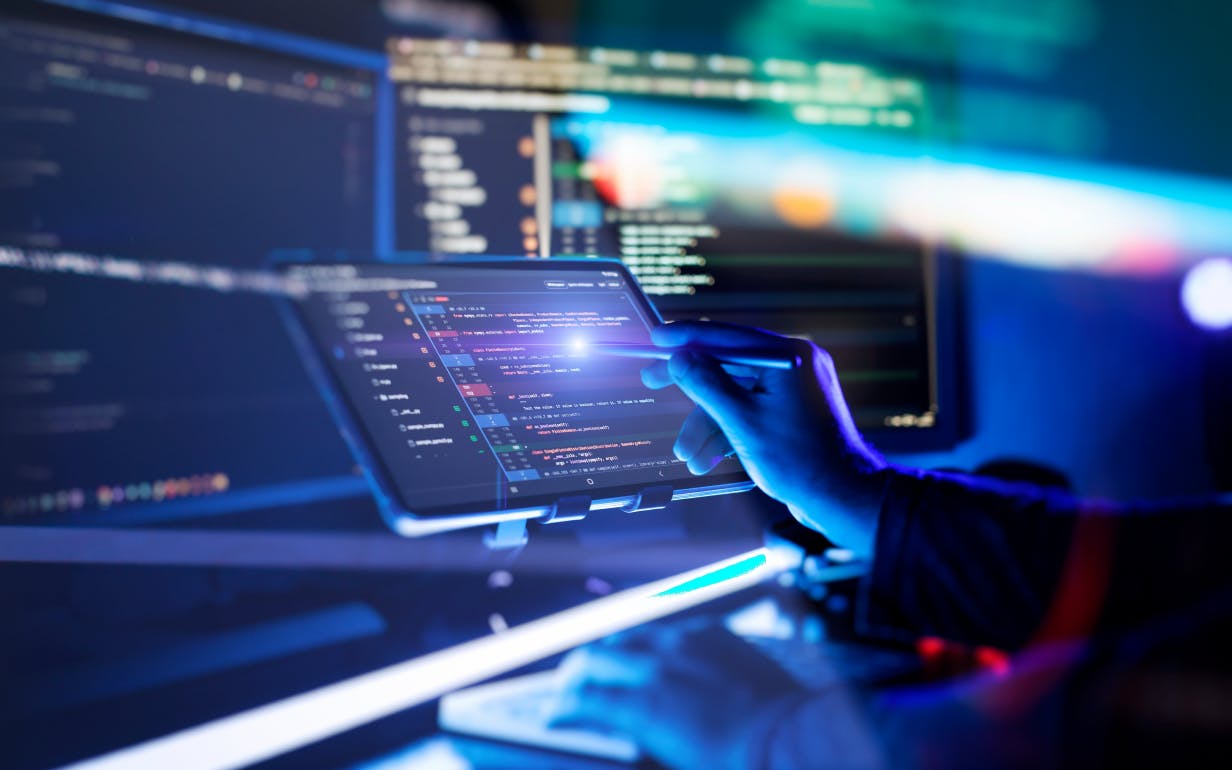
In order to become an efficient programmer, it’s essential to know how to write clean codes. This entails writing as few lines of code wherever possible and using comments and appropriate naming conventions. Why? Readability. This article will discuss the pipe ( | ) operator in Python, and how it can be used to write clean codes.
Readability is a top priority when developing an application. Programmers must code in a proper format with proper comments and indentation (albeit, not required for Python). This enables debugging and changes to be made easily, and results in satisfying the end-user and meeting business requirements.
Examples:
Task: Given an array of integers, write a program to multiply 3 with all the odd integers.
The solution is simple and illustrated as follows:
Fig 1
In the above figure, a simple for- loop is used to iterate over all the elements present in an array. While there’s no problem in the code itself, it’s not advisable to use it. Why? Look at the rules we mentioned earlier about writing clean code.
A better solution for the same task is illustrated below:
Fig 2
The code in Fig 2 is much more compact, making it a lot more readable than the previous solution. Note that the outputs are the same for both.
Here’s another solution:
Fig 3
We now have three different solutions, each distinct from the other, for a simple task. The above solution looks different because it uses the concept of pipe ( | ).
What is the pipe operation?
Pipe is a module in Python that easily connects the output from one method with the output from another method. It is a library that helps in writing cleaner code.
Before beginning with pipe, install it as shown below:
pip install pipe
Pipe functions
A few functions in the pipe library are similar to SQL commands. For example, the usage of where and select as illustrated in the figures above. Let’s discuss them in more detail.
Where
The where method helps filter out (or separate) elements in a very similar way to the where clause in SQL.
Select
The select method is similar to the map function in Python. It also has the same functionality.
Why use the where and select methods? In Fig 2 and Fig 3, both the codes are written in a single line whereas in Fig 1, there are several lines written to execute the same task. The difference between Fig 2 and Fig 3 is readability. In Fig 3, you can see that there are very few nested parentheses and hence, it’s cleaner than the code written in Fig 2.
Chain
It can be difficult to work with nested iterables, but the chain method helps serialize them and makes work much easier. The code is illustrated below:
Fig 4
Here, it’s clear that the nested list is serialized into output. Hence, it’s simpler to traverse or carry out other operations.
Traverse
As the list is not completely flattened in Fig 4, there’s another method that can be applied: the traverse method. It allows you to flatten a highly nested list, and can be used to recursively unfold iterables.
Fig 5
The above method shows how the highly nested list was flattened into a simple one-dimensional list, which was not possible using the chain method.
Grouping elements
This is useful when you have to group elements of a list into certain categories. For example, consider a list of integers that has to be divided into two groups: odd and even. You can use the groupby method to group them and then use the select method to convert them into a dictionary.
Fig 6
The groupby method works by dividing the list into two tuples in a list. The select method converts it into a list of dictionaries. Key is the first element present in the tuple, whereas the values are the second element in the tuple.
Dedup
This method is widely used to remove duplicates in a list. For example, arr=[1,2,2,3,3,4,4] is the list containing duplicate elements. On applying the dedup method, the output will be [1,2,3,4]. The set function in Python plays the same role, but the difference is that it allows a programmer to get unique elements by utilizing its key.
The following figure demonstrates it:
Fig 7
Best practices to make Python codes more readable
Here are a few best practices to employ to improve the readability of Python codes:
1. Use descriptive names for variable(s)/ function(s): Programmers must write a descriptive function/ variable name that clearly speaks about the role of that particular function or variable. This will help them understand the code more easily. For example: calculate_percentage_from_cgpa is more explanatory than just writing a function name as calculate.
2. Add a docstring: The addition of docstrings is another important practice to adhere to when writing a function. Docstrings are not comments but operate similarly to comments.
There are a few differences between them. Docstrings are written with single triple quotes (eg. '''This is a docstring’’’) whereas comments are written using the symbol # (for eg. #this is a comment). The other difference is that docstrings are not ignored by the interpreter whereas comments are. Docstrings say what the code does but not how it does it. They can be added to Python modules, functions, classes, or methods.
Look at the example below:
Fig 8
Here, the docStringDemo function is declared which has a docstring and a print statement. To show that the interpreter does not ignore the docstring, one can access the docstring of the function or a class using the , which prints the complete docstring in the output. However, this is not possible with comments. Note that indentation is important when writing a docstring otherwise it will result in IndentationError.
3. Use classes wherever possible: Classes in Python provide all features of Object Oriented Programming language. They are important because they help to bundle data into a single object which creates a new instance when called. This helps in reusability of code and hence, prevents unnecessary functions or variable declaration.
4. Keep functions short and avoid assigning them multiple tasks: It’s preferable to make a function perform a single task rather than multiple tasks as it can create confusion for other programmers. It’s also advisable to ensure that the length of a function is kept short - not more than 20 to 30 lines of code. Try using operations like pipe as described above.
5. Use proper indentation: Indentation is not such a big problem in Python compared to other languages like C++ or Java. Try not to have the indent level greater than one or two as it can affect readability.
6. Avoid multiple return statements within the same function body: This is an important point to remember as it ensures clarity of code and sustains its readability.
The methods given here showcase how easily clean codes can be written in a more optimized manner using pipe and other best practices. They’re simpler to debug and change, reduce coding hassles for programmers, and enhance processing efficiency.