How to Build Middleware for Node.js: A Complete Guide
•7 min read
- Languages, frameworks, tools, and trends
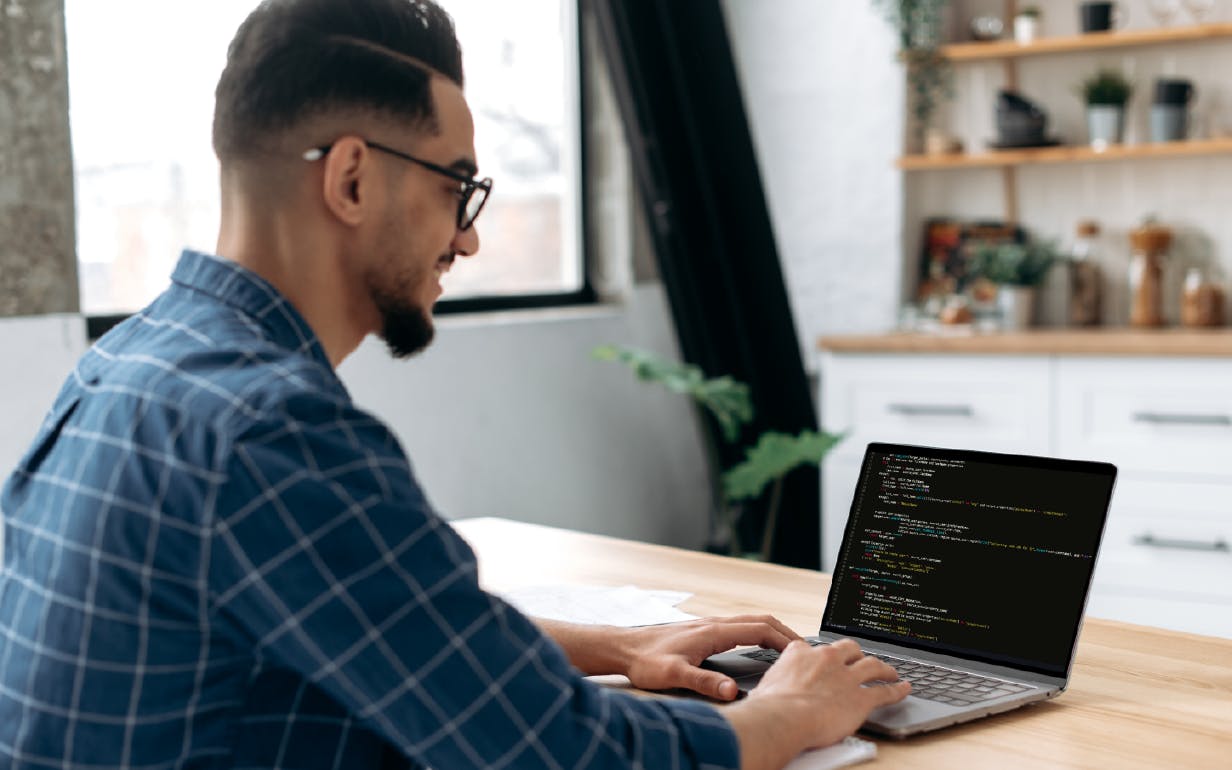
Node.js is a popular web application framework that offers easy scalability of functions and features. It also offers an easy-to-learn knowledge curve for developers. In this article, we will understand middleware in Node.js and the Express middleware.
Node.js gives developers complete freedom and privilege to develop high-performing dynamic web applications. It also helps in caching and comes with excellent and prompt community support.
Express.js, meanwhile, is an open-source backend framework for Node.js. It is an enterprise-based application and is used by the world’s leading companies. It plays a major role in tracking and preventing users from performing any specific action or recording the path of each incoming request.
The middleware in Node.js helps in making Express JS custom middleware. Middleware functions are essential when dealing with common functions in web applications.
How to create custom middleware in Node.js?
How does Node.js middleware pattern work?
Middleware comes in the middle of the request and response cycle of the node.js execution. It also provides access to many functions like request and response objects with the Next function of the cycle.
Tasks that can be performed with the middleware functions include:
- Making quick changes to the request and response objects
- Calling the next middleware immediately as per the stack
- Effectively executing any code
- Automatically terminating the request-response cycle
When the active middleware function doesn’t stop the request-response cycle, it will call the next() function to pass on the control to the next middleware function, making sure that none of the requests are pending.
What is next()?
Next() is a middleware function that calls for the control of another middleware once the code is completed. You can wait till the network operations are completed before you go to the next step. As with the functionality of route handlers, a middleware will ensure the receipt of the request and response objects effortlessly.
Now, the request object is referred to as the req variable and the response object as the res variable. The next middleware function is referred to as the next function. It plays a responsible role in creating the request-response cycle of the application.
Here are some vital tasks performed by the middleware functions:
- Completing the request and response cycle
- Executing the codes
- Calling the subsequent middleware function in the line
- Making necessary changes to the request and response objects as per the requirement
The request should not be left in the queue, especially when there is no automatic end to the request-response cycle of the current middleware function.
Middleware in Node.js
With Node.js middleware, you can run any code and modify the request and response objects. You can also call for the next middleware in the stack when the current one is completed. The example below will help you with the process of creating your Node.js middleware.
var express = require(‘require’)
var app = express()
var requestTime = function (req, res, next)
{
req.requestTime = Date.now()
next()
}
app.use(requestTime)
app.get (‘/’, function (req, res) {
var responseText = ‘Hi Familly!<br>’
responseText += ‘<small>Requested at: ‘+req.requestTime + ‘</small>’
res.send (responseText)
})
app.listen(3000)
What is Express middleware?
Middleware is an abstraction layer that works as an intermediate between the software layers. Express middleware is a function that is compiled during the lifecycle of the Express server. There is always a competition between Express and Koa for better middleware.
Every middleware can access each HTTP request and respond to every path that it is attached to. Moreover, the middleware can end the HTTP request independently or transfer it to another middleware using the next function. It will allow you to categorize the code and generate reusable middleware, just like chaining.
Types of middleware in Node.js
1. Application-level middleware
In the application-level middleware, we consider an authentication middleware and how it can be created. When the user is not authenticated, it will not be possible to call the mentioned routes. When it is necessary to build an authentication for every GET, POST call, the development of an authentication middleware will follow.
When you receive the authentication request, the authentication middleware makes progress towards the authentication code logic that is available inside it. Once the authentication is successful, the rest of the route can be called using the next function. However, when it fails, you may not be able to perform the next route as the middleware will show errors.
2. Router-level middleware
Router-level middleware is almost like the application-level middleware and works in the same way. The difference is that it can generate and limit an instance using the Express.Router() function. You can make use of the router.use() and router.METHOD() functions to load router-level middleware.
3. Build-in middleware
The build-in middleware doesn't depend on the ‘Connect’ function and unlike the previous 4.X version types, Express now acts as a module. Generally, under the Express types of middleware, you can utilize these listed middleware functions:
- json - a function that computes the incoming request by adding JSON payloads
- static - a function that acts as a static asset to the application.
4. Error-handling middleware
Express.js is capable of handling any default errors and can also define error-handling middleware functions, which are similar to the other middleware functions. The major difference is the error-handling functions.
5. Third-party middleware
Sometimes, you will need to have some additional features in the backend operations. For that, you can install the Node.js module for the specific function and then apply the same to your application (either on the application or router level).
Writing Express middleware
For generating, applying, and testing the Express middleware, you must have certain things previously installed. First, install Node and NPM using the snippet below:
npm -v && node -v
Before installing, you should check whether you are installing the right Node and NPM versions. In some cases, it will show an error so you will need to install Node again. Otherwise, you will not be able to access the required Node.js packages.
Ideally, installing Node 10.9.0 and NPM 6.4.1 should work. You can also install Node version 8 or higher and NPM versions 5 or more. When you want to test routes using any HTTP verbs except GET, install POSTman in it.
Creating middleware
Before creating the middleware in Node.js, you must install Node.js. You also have to set up an Express.js application. There are four important steps to follow:
Step 1: Structuring
Express.js middleware will have the following signature function in its snippet.
myCustomMiddleware (req,res,next)
{
// My Customized Middleware is here.
}
Req is an Express.js object which is used for requesting. Res is an object for response, and the Next function is for commanding Express.js. You need these commands to proceed to the subsequent middleware function that you have configured.
The main purpose of the middleware is to modify the req and res objects, and then compile and execute any code that is required. It also helps to terminate the request-response session and call for the next middleware in the stack.
Step 2: Modifying the req object
Modifying the req object is highly useful when you want to analyze, understand, and look for the currently logged-in user for each of their requests. For such cases, you can write a middleware similar to the one given below:
// getUserFromToken would be as per the authentication method
const getUserFromToken = require(‘../getUserFromToken”);
module.exports = function setCurrentUser (req, res, next)
{
// grab authentication token from the req header
const token = req.header(“authorization”);
// looking up the user as per the token
const user = getUserFromToken (token).then (user =>
{
// appending the user object with the requested object
req.user = user;
// calling the next middleware in the stack
next();
});
};
Once the code is complete, you can add it to your application. It is simple to execute and also enables the middleware for all the routes in your application. To enable the middleware, use the code below:
const express = require(‘express’);
const setCurrentUser = require(‘./middleware/setCurrentUser.js’);
const app = express();
app.use(setCurrentUser);
//
Step 3: Modifying the res object
With the response object, you can easily set a custom header for all your response objects. This is quite easy with a middleware code like the one below:
module.exports = function addGatorHeader (req, res, next)
{
res.setHeader (“X-Gator-policy”, “Chomp-Chomp”);
next();
};
Step 4: Ending the cycle
The most common function of middleware is validating whether the user object is properly added to your request object. At the end of the execution, if you don’t get the desired output or can’t find the user, you should terminate the request/response cycle. To validate this approach, you can use the below-given code as an example:
module.exports = function isLoggedIn(req, res, next)
{
if(req.user)
{
// user will be authenticated
next();
}
else
{
// return unauthorized user
res.send (401, ‘Unauthorized’);
}
};
The final step is to add the middleware efficiently to your application, where ordering becomes more crucial. Below is an example that will support the previous approach. You can use a new middleware to the existing application and create an authentication while using a single route.
The coding for the server.js will be as follows:
const express = require (“express”);
const setCurrentUser = require (“./middleware/setCurrentUser.js”);
const isLoggedIn = require (“./middleware/isLoggedIn.js”);
const app = express ();
app.use (setCurrentUser);
app.get (“/users”, isLoggedIn, function (req, res)
{
// getting users
});
In the above example, only the user’s path will expect the user to be authenticated.
The syntaxes provided here should help you get a handle on the use of Express middleware in Node.js. With the aid of the use-cases mentioned, you should be able to confidently use Express middleware, even if you are new to it.
FAQs:
1. Why is middleware needed?
Middleware is required for helping developers in building applications more effectively and efficiently. The middleware will act as a connection between data, applications, and the users. When you are an organization with a multi-cloud environment, then middleware will make it more cost-effective for the development and running of the application at scale.
2. What is middleware in Node.js?
The middleware in node.js is a function that will have all the access for requesting an object, responding to an object, and moving to the next middleware function in the application request-response cycle. This function can be used for modifying the req and res objects for tasks like adding response headers, parsing requesting bodies, and so on.